Material-UI (MUI) trong React
Material-UI (MUI) là một thư viện giao diện người dùng phổ biến cho React, tuân theo thiết kế Material Design của Google. Với MUI, bạn có thể nhanh chóng xây dựng các ứng dụng React có giao diện đẹp mắt, nhất quán và thân thiện với người dùng.
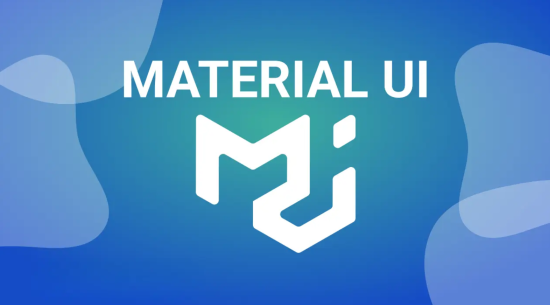
1. Material-UI là gì?
Material-UI (MUI) là một thư viện UI components được xây dựng dành riêng cho React. Nó cung cấp một bộ sưu tập các component sẵn có, được thiết kế theo nguyên tắc Material Design.
Tính năng nổi bật của Material-UI:
- Component sẵn có: Nhiều component UI như Button, Dialog, Form, Table,...
- Dễ dàng tùy chỉnh: Có thể override và theme dễ dàng.
- Hỗ trợ responsive: Tích hợp hệ thống grid và breakpoints mạnh mẽ.
- Accessibility: Tuân theo tiêu chuẩn ARIA để hỗ trợ người dùng khuyết tật.
2. Cài đặt Material-UI trong React
2.1. Cài đặt thư viện MUI
Chạy lệnh sau trong terminal:
npm install @mui/material @emotion/react @emotion/styled
- @mui/material: Thư viện component Material-UI.
- @emotion/react & @emotion/styled: Công cụ hỗ trợ styled components cho MUI.
2.2. Cài đặt Icons (tuỳ chọn)
npm install @mui/icons-material
2.3. Cấu hình cơ bản
Trong index.js
hoặc App.js
, bọc ứng dụng với ThemeProvider:
import React from 'react';
import ReactDOM from 'react-dom/client';
import { ThemeProvider, createTheme } from '@mui/material/styles';
import App from './App';
const theme = createTheme({
palette: {
primary: {
main: '#1976d2',
},
secondary: {
main: '#dc004e',
},
},
});
ReactDOM.createRoot(document.getElementById('root')).render(
<ThemeProvider theme={theme}>
<App />
</ThemeProvider>
);
3. Sử dụng Material-UI Components
3.1. Button (Nút bấm)
import Button from '@mui/material/Button';
function App() {
return (
<div>
<Button variant="contained" color="primary">
Primary Button
</Button>
<Button variant="outlined" color="secondary">
Outlined Button
</Button>
</div>
);
}
export default App;
Giải thích:
-
variant="contained"
: Nút có nền màu. -
variant="outlined"
: Nút chỉ có viền. -
color
: Xác định màu sắc của nút (primary
,secondary
,success
,...).
3.2. Typography (Kiểu chữ)
import Typography from '@mui/material/Typography';
function App() {
return (
<div>
<Typography variant="h1">Heading 1</Typography>
<Typography variant="body1">Body text example</Typography>
</div>
);
}
Giải thích:
-
variant
: Xác định loại văn bản (h1
,h2
,body1
,body2
). -
color
: Chọn màu chữ (primary
,secondary
,textPrimary
).
3.3. TextField (Trường nhập liệu)
import TextField from '@mui/material/TextField';
function App() {
return (
<div>
<TextField label="Username" variant="outlined" />
<TextField label="Password" variant="filled" type="password" />
</div>
);
}
Giải thích:
-
label
: Tiêu đề của ô nhập liệu. -
variant
: Kiểu của ô nhập (outlined
,filled
,standard
). -
type
: Loại dữ liệu (text
,password
).
3.4. Card (Thẻ nội dung)
import Card from '@mui/material/Card';
import CardContent from '@mui/material/CardContent';
import Typography from '@mui/material/Typography';
function App() {
return (
<Card>
<CardContent>
<Typography variant="h5">Card Title</Typography>
<Typography variant="body2">This is a card description.</Typography>
</CardContent>
</Card>
);
}
Giải thích:
-
Card
: Tạo thẻ nội dung. -
CardContent
: Phần nội dung chính của thẻ.
3.5. Grid System (Hệ thống lưới)
import Grid from '@mui/material/Grid';
import Paper from '@mui/material/Paper';
function App() {
return (
<Grid container spacing={2}>
<Grid item xs={12} sm={6}>
<Paper>Item 1</Paper>
</Grid>
<Grid item xs={12} sm={6}>
<Paper>Item 2</Paper>
</Grid>
</Grid>
);
}
Giải thích:
-
container
: Tạo lưới chứa. -
item
: Tạo các ô trong lưới. -
spacing
: Khoảng cách giữa các ô. -
xs
,sm
: Breakpoints responsive.
4. Tạo Theme tùy chỉnh
Trong file ThemeProvider, bạn có thể tạo theme riêng:
import { createTheme } from '@mui/material/styles';
const theme = createTheme({
palette: {
primary: {
main: '#4caf50',
},
secondary: {
main: '#ff5722',
},
},
typography: {
fontFamily: 'Roboto, Arial, sans-serif',
h1: {
fontSize: '2rem',
},
},
});
5. So sánh Material-UI và Tailwind CSS
Material-UI | Tailwind CSS |
---|---|
Dùng component dựng sẵn | Class tiện ích (utility-first) |
Phù hợp với dự án nhanh | Tuỳ chỉnh tự do hơn |
Theme cấu hình rõ ràng | Cấu hình qua file config |
Học curve thấp | Học curve cao hơn |
6. Ưu và Nhược điểm của Material-UI
Ưu điểm:
- Bộ component đa dạng và đẹp mắt.
- Hỗ trợ tùy chỉnh theme dễ dàng.
- Thân thiện với responsive design.
- Hỗ trợ accessibility.
Nhược điểm:
- Bundle size lớn nếu không tree-shaking tốt.
- Tùy chỉnh sâu có thể phức tạp.
7. Kết luận
Material-UI (MUI) là một công cụ mạnh mẽ cho các dự án React, giúp bạn xây dựng giao diện đẹp mắt, nhất quán và dễ bảo trì. Với hệ thống theme mạnh mẽ và các component đa dạng, MUI là lựa chọn hoàn hảo cho cả dự án nhỏ lẫn lớn.
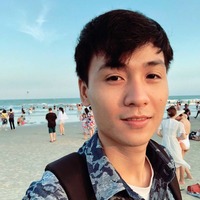
Với hơn 10 năm kinh nghiệm lập trình web và từng làm việc với nhiều framework, ngôn ngữ như PHP, JavaScript, React, jQuery, CSS, HTML, CakePHP, Laravel..., tôi hy vọng những kiến thức được chia sẻ tại đây sẽ hữu ích và thiết thực cho các bạn.
Xem thêm
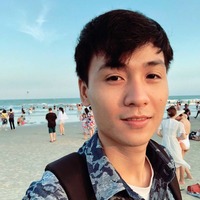
Chào, tôi là Vũ. Đây là blog hướng dẫn lập trình của tôi.
Liên hệ công việc qua email dưới đây.
lhvuctu@gmail.com