Form Validation – Kiểm tra dữ liệu đầu vào
Khi xây dựng một ứng dụng web, việc xác thực dữ liệu đầu vào là rất quan trọng để đảm bảo tính bảo mật và tính toàn vẹn của dữ liệu. CodeIgniter cung cấp một hệ thống Form Validation mạnh mẽ giúp lập trình viên kiểm tra dữ liệu một cách dễ dàng trước khi lưu vào cơ sở dữ liệu.
Mục tiêu bài học:
- Hiểu cách hoạt động của Form Validation trong CodeIgniter.
- Biết cách sử dụng Rule Sets để xác thực dữ liệu đầu vào.
- Hiển thị lỗi validation trong giao diện (View).
- Xây dựng hệ thống xác thực nâng cao.
- Thực hành tạo Form đăng ký tài khoản với các quy tắc kiểm tra dữ liệu hợp lệ.

Form Validation
1. Giới thiệu về Form Validation trong CodeIgniter
CodeIgniter cung cấp Form Validation Library, cho phép kiểm tra dữ liệu đầu vào của người dùng bằng cách định nghĩa các quy tắc (Rule Sets) như:
- Kiểm tra dữ liệu không được để trống (
required
). - Kiểm tra định dạng email (
valid_email
). - Kiểm tra độ dài ký tự (
min_length
,max_length
). - Kiểm tra dữ liệu có trùng trong cơ sở dữ liệu không (
is_unique
).
Nạp thư viện Form Validation
Trong CodeIgniter 4:
use CodeIgniter\Validation\Validation;
Trong CodeIgniter 3:
$this->load->library('form_validation');
2. Xác thực dữ liệu đầu vào với Rule Sets
Ví dụ về Rule Sets trong CodeIgniter 4
$validationRules = [
'username' => 'required|min_length[5]|max_length[20]|alpha_numeric',
'email' => 'required|valid_email|is_unique[users.email]',
'password' => 'required|min_length[8]',
];
if (!$this->validate($validationRules)) {
return redirect()->back()->withInput()->with('errors', $this->validator->getErrors());
}
Giải thích các Rule Sets:
-
required
: Bắt buộc nhập. -
min_length[5]
: Độ dài tối thiểu là 5 ký tự. -
max_length[20]
: Độ dài tối đa là 20 ký tự. -
alpha_numeric
: Chỉ chứa chữ cái và số. -
valid_email
: Kiểm tra email hợp lệ. -
is_unique[users.email]
: Kiểm tra email đã tồn tại trong bảngusers
hay chưa.
3. Hiển thị lỗi Validation trong View
Trong Controller, truyền lỗi validation sang View
$data['validation'] = $this->validator;
return view('register', $data);
Trong View (register.php
), hiển thị lỗi validation
<?php if (isset($validation)) : ?>
<div class="alert alert-danger">
<?= $validation->listErrors(); ?>
</div>
<?php endif; ?>
Cách hiển thị lỗi của từng trường cụ thể:
<?= $validation->showError('email'); ?>
4. Xây dựng hệ thống xác thực nâng cao
Tạo Rule Sets trong File Riêng (CodeIgniter 4)
Tạo file app/Config/Validation.php
để quản lý tập trung các Rule Sets:
<?php
namespace Config;
class Validation
{
public $register = [
'username' => 'required|min_length[5]|max_length[20]|alpha_numeric',
'email' => 'required|valid_email|is_unique[users.email]',
'password' => 'required|min_length[8]',
];
}
Gọi Rule Sets trong Controller
if (!$this->validate(config('Validation')->register)) {
return redirect()->back()->withInput()->with('errors', $this->validator->getErrors());
}
5. Thực hành: Xây dựng Form đăng ký tài khoản
Bước 1: Tạo View Form Đăng Ký (app/Views/register.php
)
<form action="<?= base_url('register/process'); ?>" method="post">
<label>Username:</label>
<input type="text" name="username" value="<?= old('username'); ?>">
<?= session('errors.username'); ?>
<label>Email:</label>
<input type="email" name="email" value="<?= old('email'); ?>">
<?= session('errors.email'); ?>
<label>Password:</label>
<input type="password" name="password">
<?= session('errors.password'); ?>
<button type="submit">Đăng ký</button>
</form>
Bước 2: Tạo Controller Xử Lý Đăng Ký (app/Controllers/Register.php
)
<?php
namespace App\Controllers;
use App\Models\UserModel;
use CodeIgniter\Controller;
class Register extends Controller
{
public function index()
{
return view('register');
}
public function process()
{
$validationRules = [
'username' => 'required|min_length[5]|max_length[20]|alpha_numeric',
'email' => 'required|valid_email|is_unique[users.email]',
'password' => 'required|min_length[8]',
];
if (!$this->validate($validationRules)) {
return redirect()->back()->withInput()->with('errors', $this->validator->getErrors());
}
// Lưu dữ liệu vào database
$userModel = new UserModel();
$userModel->save([
'username' => $this->request->getPost('username'),
'email' => $this->request->getPost('email'),
'password' => password_hash($this->request->getPost('password'), PASSWORD_DEFAULT),
]);
return redirect()->to('/login')->with('success', 'Đăng ký thành công!');
}
}
Bước 3: Tạo Model User (app/Models/UserModel.php
)
<?php
namespace App\Models;
use CodeIgniter\Model;
class UserModel extends Model
{
protected $table = 'users';
protected $allowedFields = ['username', 'email', 'password'];
}
Kết luận
Tóm tắt nội dung chính:
- CodeIgniter cung cấp thư viện Form Validation để kiểm tra dữ liệu đầu vào.
-
Rule Sets giúp xác thực dữ liệu với nhiều điều kiện như
required
,valid_email
,min_length
,is_unique
. - Có thể hiển thị lỗi validation trong View để thông báo cho người dùng.
- Xây dựng hệ thống xác thực nâng cao bằng cách quản lý Rule Sets tập trung.
- Thực hành tạo form đăng ký tài khoản với các quy tắc kiểm tra dữ liệu hợp lệ.
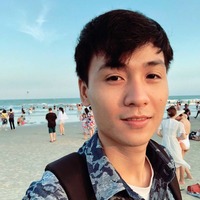
Với hơn 10 năm kinh nghiệm lập trình web và từng làm việc với nhiều framework, ngôn ngữ như PHP, JavaScript, React, jQuery, CSS, HTML, CakePHP, Laravel..., tôi hy vọng những kiến thức được chia sẻ tại đây sẽ hữu ích và thiết thực cho các bạn.
Xem thêm
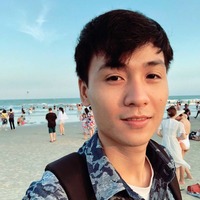
Chào, tôi là Vũ. Đây là blog hướng dẫn lập trình của tôi.
Liên hệ công việc qua email dưới đây.
lhvuctu@gmail.com