Models – Tương tác với cơ sở dữ liệu
Model là thành phần quan trọng trong kiến trúc MVC của CodeIgniter, giúp chúng ta làm việc với cơ sở dữ liệu một cách hiệu quả. Trong bài học này, chúng ta sẽ tìm hiểu cách sử dụng Model để kết nối với cơ sở dữ liệu MySQL và thực hiện các thao tác CRUD (Create, Read, Update, Delete).
Mục tiêu bài học:
- Hiểu khái niệm Model trong CodeIgniter.
- Kết nối với cơ sở dữ liệu MySQL.
- Thực hiện CRUD với Model.
- Sử dụng Query Builder để tối ưu truy vấn.
- Thực hành xây dựng chức năng quản lý danh sách người dùng.
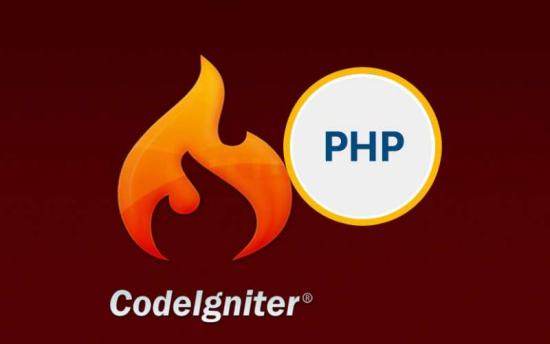
MODELS
1. Giới thiệu về Model trong CodeIgniter
Model là gì?
Model là thành phần chịu trách nhiệm tương tác với cơ sở dữ liệu trong ứng dụng CodeIgniter. Nó giúp chúng ta thực hiện các thao tác như: lấy dữ liệu, thêm mới, cập nhật và xóa dữ liệu.
Nơi lưu trữ Model trong CodeIgniter 4:
Tất cả các Model nằm trong thư mục:
app/Models/
2. Kết nối với cơ sở dữ liệu MySQL
Cấu hình kết nối trong CodeIgniter 4
Mở file cấu hình .env
và chỉnh sửa thông tin kết nối:
database.default.hostname = localhost
database.default.database = my_database
database.default.username = root
database.default.password =
database.default.DBDriver = MySQLi
Tạo cơ sở dữ liệu và bảng users
trong MySQL
Chạy lệnh SQL sau để tạo bảng users
:
CREATE TABLE users (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(100) NOT NULL,
email VARCHAR(100) NOT NULL UNIQUE,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
3. Tạo Model trong CodeIgniter
Tạo file UserModel.php
trong thư mục app/Models/
<?php
namespace App\Models;
use CodeIgniter\Model;
class UserModel extends Model
{
protected $table = 'users'; // Tên bảng
protected $primaryKey = 'id'; // Khóa chính
protected $allowedFields = ['name', 'email']; // Các cột được phép thao tác
}
Giải thích:
-
$table
: Chỉ định bảngusers
. -
$primaryKey
: Xác định cộtid
làm khóa chính. -
$allowedFields
: Chỉ định các trường có thể insert/update.
4. Thực hiện CRUD với Model
Thêm người dùng (Create)
Thêm dữ liệu vào bảng users
$userModel = new \App\Models\UserModel();
$userModel->insert([
'name' => 'Nguyễn Văn A',
'email' => 'nguyenvana@example.com'
]);
Lấy danh sách người dùng (Read)
Lấy tất cả người dùng
$userModel = new \App\Models\UserModel();
$users = $userModel->findAll();
Lấy một người dùng theo ID
$user = $userModel->find(1);
Cập nhật thông tin người dùng (Update)
Cập nhật tên của người dùng có ID = 1
$userModel->update(1, ['name' => 'Trần Văn B']);
Xóa người dùng (Delete)
Xóa người dùng có ID = 1
$userModel->delete(1);
5. Sử dụng Query Builder để tối ưu truy vấn
Query Builder giúp tạo truy vấn SQL một cách dễ dàng và an toàn.
Lấy danh sách người dùng có email chứa "gmail"
$users = $userModel->like('email', 'gmail')->findAll();
Lấy danh sách người dùng, sắp xếp theo created_at
$users = $userModel->orderBy('created_at', 'DESC')->findAll();
6. Thực hành: Xây dựng chức năng quản lý danh sách người dùng
Tạo Controller UserController.php
Mở app/Controllers/UserController.php
và tạo Controller
<?php
namespace App\Controllers;
use App\Models\UserModel;
class UserController extends BaseController
{
public function index()
{
$userModel = new UserModel();
$data['users'] = $userModel->findAll();
return view('users/index', $data);
}
public function create()
{
return view('users/create');
}
public function store()
{
$userModel = new UserModel();
$userModel->insert([
'name' => $this->request->getPost('name'),
'email' => $this->request->getPost('email')
]);
return redirect()->to('/users');
}
}
Tạo View hiển thị danh sách người dùng
Tạo file app/Views/users/index.php
<h2>Danh sách người dùng</h2>
<table border="1">
<tr>
<th>ID</th>
<th>Tên</th>
<th>Email</th>
</tr>
<?php foreach ($users as $user): ?>
<tr>
<td><?= $user['id']; ?></td>
<td><?= $user['name']; ?></td>
<td><?= $user['email']; ?></td>
</tr>
<?php endforeach; ?>
</table>
<a href="/users/create">Thêm người dùng</a>
Tạo Form thêm người dùng
Tạo file app/Views/users/create.php
<h2>Thêm người dùng</h2>
<form action="/users/store" method="post">
<label for="name">Tên:</label>
<input type="text" name="name" required>
<label for="email">Email:</label>
<input type="email" name="email" required>
<button type="submit">Lưu</button>
</form>
Cấu hình Routing cho chức năng quản lý người dùng
Thêm vào app/Config/Routes.php
$routes->get('users', 'UserController::index');
$routes->get('users/create', 'UserController::create');
$routes->post('users/store', 'UserController::store');
-
Khi truy cập
/users
, danh sách người dùng sẽ hiển thị. -
Khi truy cập
/users/create
, form thêm người dùng sẽ xuất hiện.
Kết luận
- Model giúp tương tác với cơ sở dữ liệu một cách dễ dàng.
-
Kết nối MySQL bằng CodeIgniter rất đơn giản với file cấu hình
.env
. -
Thực hiện CRUD với Model bằng các phương thức
insert()
,find()
,update()
,delete()
. - Query Builder giúp tối ưu truy vấn và tăng tính bảo mật.
- Thực hành giúp xây dựng chức năng quản lý danh sách người dùng.
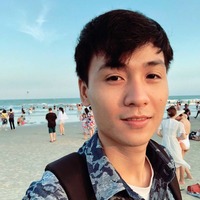
Với hơn 10 năm kinh nghiệm lập trình web và từng làm việc với nhiều framework, ngôn ngữ như PHP, JavaScript, React, jQuery, CSS, HTML, CakePHP, Laravel..., tôi hy vọng những kiến thức được chia sẻ tại đây sẽ hữu ích và thiết thực cho các bạn.
Xem thêm
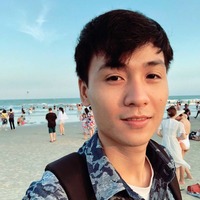
Chào, tôi là Vũ. Đây là blog hướng dẫn lập trình của tôi.
Liên hệ công việc qua email dưới đây.
lhvuctu@gmail.com