Component Lifecycle Hooks trong Vue.js
Mỗi Component trong Vue.js có vòng đời (Lifecycle), bắt đầu từ lúc được khởi tạo đến khi bị hủy bỏ. Vue cung cấp các Lifecycle Hooks giúp chúng ta can thiệp vào các giai đoạn khác nhau trong vòng đời của Component.
Mục tiêu bài học:
- Hiểu Lifecycle Hooks là gì và cách hoạt động của chúng.
- Nắm rõ các giai đoạn quan trọng của vòng đời Component.
- Ứng dụng thực tế của Lifecycle Hooks trong việc lấy dữ liệu, cập nhật giao diện, dọn dẹp tài nguyên.
-
Thực hành: Tạo một Component hiển thị thời gian thực, cập nhật mỗi giây với
mounted()
, và dừng khi Component bị hủy vớidestroyed()
.
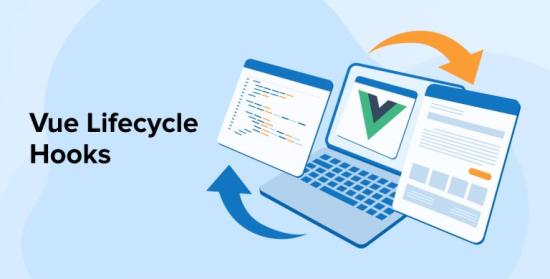
Component Lifecycle Hooks
1. Lifecycle Hooks là gì?
Lifecycle Hooks là các hàm đặc biệt của Vue.js, được gọi tại từng giai đoạn khác nhau trong vòng đời của Component. Chúng giúp chúng ta thực hiện các hành động như:
- Lấy dữ liệu từ API khi Component vừa khởi tạo.
- Ghi log hoặc theo dõi dữ liệu khi Component thay đổi.
- Dọn dẹp bộ nhớ khi Component bị hủy.
2. Các giai đoạn của Component trong Vue.js
Mỗi Component trong Vue có các giai đoạn chính sau:
Giai đoạn | Hook | Mô tả |
---|---|---|
Khởi tạo | beforeCreate() |
Chưa có dữ liệu, chưa khởi tạo instance. |
created() |
Dữ liệu đã có, nhưng chưa render ra UI. | |
Gắn vào DOM | beforeMount() |
HTML template đã biên dịch nhưng chưa hiển thị trên UI. |
mounted() |
Component hiển thị trên UI, DOM đã sẵn sàng. (Thường dùng để gọi API, setInterval, event listener) | |
Cập nhật dữ liệu | beforeUpdate() |
Dữ liệu thay đổi nhưng chưa cập nhật lên UI. |
updated() |
UI đã cập nhật với dữ liệu mới. | |
Hủy bỏ Component | beforeUnmount() |
Component chuẩn bị bị xóa khỏi DOM. |
unmounted() |
Component đã bị xóa hoàn toàn. (Dọn dẹp sự kiện, xóa timer, hủy API request) |
Hình minh họa vòng đời Component
beforeCreate → created → beforeMount → mounted → beforeUpdate → updated → beforeUnmount → unmounted
3. Ứng dụng thực tế của Lifecycle Hooks
Trường hợp sử dụng phổ biến:
- Lấy dữ liệu từ API khi Component được mounted().
- Ghi log thay đổi dữ liệu trong updated().
- Dọn dẹp bộ nhớ (xóa timer, hủy API request) khi Component unmounted().
Ví dụ: Gọi API khi Component được mounted
mounted() {
fetch("https://jsonplaceholder.typicode.com/users")
.then(response => response.json())
.then(data => {
this.users = data;
});
}
Ví dụ: Dọn dẹp timer khi Component bị hủy
unmounted() {
clearInterval(this.timer);
}
Thực hành: Tạo Component hiển thị thời gian thực
Yêu cầu:
- Tạo một Component hiển thị giờ hiện tại và cập nhật mỗi giây bằng
mounted()
. - Khi Component bị hủy, dừng đồng hồ bằng
unmounted()
.
Bước 1: Cấu trúc thư mục
/project
/index.html
/main.js
/ClockComponent.vue
Bước 2: Tạo file index.html
<!DOCTYPE html>
<html lang="vi">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Clock Component</title>
</head>
<body>
<div id="app"></div>
<script type="module" src="main.js"></script>
</body>
</html>
Bước 3: Tạo Component ClockComponent.vue
const ClockComponent = {
data() {
return {
time: new Date().toLocaleTimeString(),
timer: null
};
},
template: `
<div>
<h2>Giờ hiện tại: {{ time }}</h2>
<button @click="$emit('stop-clock')">Dừng đồng hồ</button>
</div>
`,
methods: {
updateClock() {
this.time = new Date().toLocaleTimeString();
}
},
mounted() {
console.log("ClockComponent đã mounted!");
this.timer = setInterval(this.updateClock, 1000);
},
unmounted() {
console.log("ClockComponent đã unmounted! Dừng đồng hồ...");
clearInterval(this.timer);
}
};
Bước 4: Tạo main.js
(Component cha điều khiển đồng hồ)
import { createApp } from "vue";
import ClockComponent from "./ClockComponent.vue";
const app = createApp({
components: {
"clock-component": ClockComponent
},
data() {
return {
showClock: true
};
},
template: `
<div>
<h1>Vue.js Lifecycle Hooks</h1>
<button @click="showClock = !showClock">
{{ showClock ? "Ẩn đồng hồ" : "Hiển thị đồng hồ" }}
</button>
<clock-component v-if="showClock" @stop-clock="showClock = false"></clock-component>
</div>
`
});
app.mount("#app");
Kết luận
Tóm tắt kiến thức quan trọng:
- Lifecycle Hooks giúp kiểm soát vòng đời của Component.
-
mounted()
thường được dùng để lấy dữ liệu hoặc khởi tạo timer. -
unmounted()
dùng để dọn dẹp tài nguyên, xóa event listener hoặc timer. -
Thực hành: Tạo đồng hồ cập nhật thời gian thực với
mounted()
và dừng khiunmounted()
.
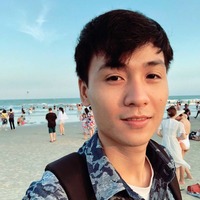
Với hơn 10 năm kinh nghiệm lập trình web và từng làm việc với nhiều framework, ngôn ngữ như PHP, JavaScript, React, jQuery, CSS, HTML, CakePHP, Laravel..., tôi hy vọng những kiến thức được chia sẻ tại đây sẽ hữu ích và thiết thực cho các bạn.
Xem thêm
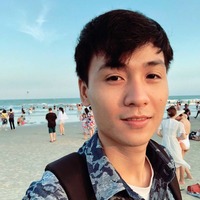
Chào, tôi là Vũ. Đây là blog hướng dẫn lập trình của tôi.
Liên hệ công việc qua email dưới đây.
lhvuctu@gmail.com