Route Parameters và Query Params
Trong Angular, Route Parameters và Query Params là hai cách chính để truyền dữ liệu giữa các component thông qua URL.
-
Route Parameters: Dùng để truyền dữ liệu cố định trong URL, thường dùng để hiển thị chi tiết một đối tượng (ví dụ:
/products/1
). -
Query Params: Dùng để truyền dữ liệu động mà không ảnh hưởng đến cấu trúc route, thường dùng cho bộ lọc, phân trang (ví dụ:
/products?page=2&sort=asc
).
Mục tiêu bài học:
- Hiểu Route Parameters và cách sử dụng.
- Sử dụng
ActivatedRoute
để lấy dữ liệu từ URL. - Hiểu Query Params và cách truyền dữ liệu linh hoạt.
- Lắng nghe sự thay đổi của Route Parameters bằng
snapshot
vàobservable
.
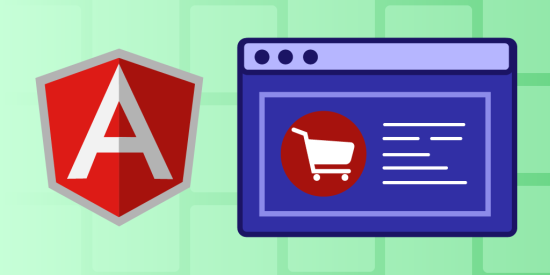
Route Parameters và Query Params trong Angular
1. Truyền và nhận tham số từ URL (Route Parameters)
Bước 1: Cấu hình route có tham số trong app-routing.module.ts
.
import { NgModule } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
import { ProductListComponent } from './product-list/product-list.component';
import { ProductDetailComponent } from './product-detail/product-detail.component';
const routes: Routes = [
{ path: 'products', component: ProductListComponent }, // Danh sách sản phẩm
{ path: 'products/:id', component: ProductDetailComponent } // Chi tiết sản phẩm
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
Bước 2: Điều hướng đến URL có tham số trong product-list.component.html
.
<ul>
<li *ngFor="let product of products">
<a [routerLink]="['/products', product.id]">{{ product.name }}</a>
</li>
</ul>
Bước 3: Lấy tham số từ URL trong product-detail.component.ts
.
import { Component, OnInit } from '@angular/core';
import { ActivatedRoute } from '@angular/router';
@Component({
selector: 'app-product-detail',
templateUrl: './product-detail.component.html',
styleUrls: ['./product-detail.component.css']
})
export class ProductDetailComponent implements OnInit {
productId: string | null = '';
constructor(private route: ActivatedRoute) {}
ngOnInit() {
this.productId = this.route.snapshot.paramMap.get('id'); // Lấy tham số từ URL
}
}
Giải thích:
-
path: 'products/:id'
→ Định nghĩa route với tham sốid
. -
[routerLink]="['/products', product.id]"
→ Điều hướng đếnproducts/:id
. -
this.route.snapshot.paramMap.get('id')
→ Lấy giá trịid
từ URL.
2. Lắng nghe sự thay đổi của Route Parameters bằng Observable
Nếu tham số thay đổi trong cùng một component (ví dụ: người dùng chuyển từ /products/1
sang /products/2
), ta cần sử dụng Observable để theo dõi sự thay đổi.
Cập nhật product-detail.component.ts
:
import { Component, OnInit } from '@angular/core';
import { ActivatedRoute } from '@angular/router';
@Component({
selector: 'app-product-detail',
templateUrl: './product-detail.component.html',
styleUrls: ['./product-detail.component.css']
})
export class ProductDetailComponent implements OnInit {
productId: string | null = '';
constructor(private route: ActivatedRoute) {}
ngOnInit() {
this.route.paramMap.subscribe(params => {
this.productId = params.get('id');
});
}
}
Giải thích:
-
route.paramMap.subscribe()
→ Lắng nghe sự thay đổi của tham sốid
trong URL. - Khi người dùng nhấn vào một sản phẩm khác,
productId
sẽ được cập nhật mà không cần tải lại trang.
3. Sử dụng Query Params để truyền dữ liệu động
Bước 1: Cấu hình điều hướng với Query Params trong product-list.component.html
.
<button [routerLink]="['/products']" [queryParams]="{ page: 1, sort: 'asc' }">
Xem sản phẩm theo trang 1, sắp xếp tăng dần
</button>
Bước 2: Lấy Query Params trong product-list.component.ts
.
import { Component, OnInit } from '@angular/core';
import { ActivatedRoute } from '@angular/router';
@Component({
selector: 'app-product-list',
templateUrl: './product-list.component.html',
styleUrls: ['./product-list.component.css']
})
export class ProductListComponent implements OnInit {
page: number = 1;
sort: string = 'asc';
constructor(private route: ActivatedRoute) {}
ngOnInit() {
this.route.queryParamMap.subscribe(params => {
this.page = Number(params.get('page')) || 1;
this.sort = params.get('sort') || 'asc';
});
}
}
Giải thích:
-
[queryParams]
→ Truyền tham số vào URL dưới dạng?page=1&sort=asc
. -
route.queryParamMap.subscribe()
→ Lắng nghe sự thay đổi của Query Params.
4. Điều hướng với Query Params bằng TypeScript
Ngoài [queryParams]
, ta có thể sử dụng router.navigate()
để điều hướng bằng TypeScript.
Ví dụ trong product-list.component.ts
:
import { Component } from '@angular/core';
import { Router } from '@angular/router';
@Component({
selector: 'app-product-list',
templateUrl: './product-list.component.html',
styleUrls: ['./product-list.component.css']
})
export class ProductListComponent {
constructor(private router: Router) {}
filterProducts() {
this.router.navigate(['/products'], { queryParams: { page: 2, sort: 'desc' } });
}
}
Trong HTML:
<button (click)="filterProducts()">Xem trang 2, sắp xếp giảm dần</button>
Giải thích:
-
router.navigate(['/products'], { queryParams: { page: 2, sort: 'desc' } })
→ Điều hướng và truyền Query Params bằng TypeScript.
Kết luận
Nội dung | Mô tả |
---|---|
Route Parameters | Truyền dữ liệu cố định trong URL, ví dụ: /products/1 . |
Query Params | Truyền dữ liệu động trong URL, ví dụ: /products?page=2&sort=asc . |
ActivatedRoute |
Dùng để lấy Route Parameters và Query Params từ URL. |
Snapshot vs Observable | Snapshot lấy dữ liệu một lần, Observable lắng nghe thay đổi liên tục. |
Điều hướng bằng router.navigate() |
Điều hướng linh hoạt bằng TypeScript. |
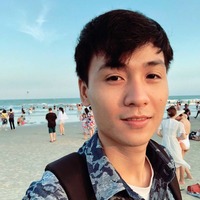
Với hơn 10 năm kinh nghiệm lập trình web và từng làm việc với nhiều framework, ngôn ngữ như PHP, JavaScript, React, jQuery, CSS, HTML, CakePHP, Laravel..., tôi hy vọng những kiến thức được chia sẻ tại đây sẽ hữu ích và thiết thực cho các bạn.
Xem thêm
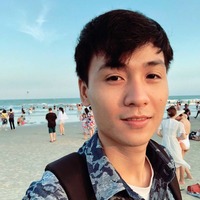
Chào, tôi là Vũ. Đây là blog hướng dẫn lập trình của tôi.
Liên hệ công việc qua email dưới đây.
lhvuctu@gmail.com