Tạo và sử dụng Services trong Angular
Trong Angular, Service đóng vai trò quan trọng trong việc quản lý logic và dữ liệu, giúp tách biệt logic xử lý khỏi Component để tăng tính tái sử dụng và bảo trì. Service kết hợp với Dependency Injection (DI) giúp ứng dụng Angular hoạt động hiệu quả hơn.
Mục tiêu bài học:
- Hiểu Service là gì và tại sao cần sử dụng Service trong Angular.
- Biết cách tạo một Service bằng Angular CLI.
- Biết cách sử dụng Service trong Component.
- Biết cách chia sẻ dữ liệu giữa các Component bằng Service.
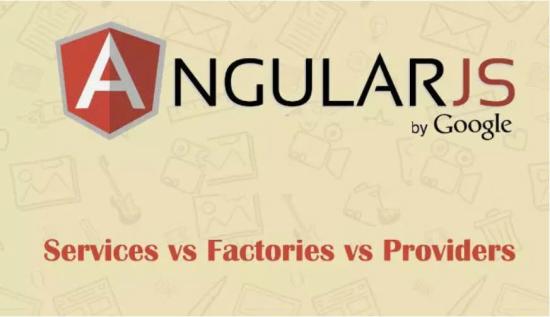
Tạo và sử dụng Services
1. Giới thiệu về Services và vai trò của chúng
Service là gì?
-
Service trong Angular là một lớp (
class
) chứa logic xử lý và dữ liệu, có thể được sử dụng lại trong nhiều Component. - Service giúp tách biệt business logic khỏi Component, giúp Component chỉ tập trung vào việc hiển thị dữ liệu.
Lợi ích của Service:
- Tái sử dụng: Một Service có thể được dùng trong nhiều Component.
- Dễ bảo trì: Tách riêng logic xử lý giúp dễ sửa đổi khi cần.
- Giảm lặp code: Thay vì viết lại logic trong nhiều Component, chỉ cần gọi Service.
- Quản lý dữ liệu chung: Giúp chia sẻ dữ liệu giữa các Component.
2. Tạo Service bằng Angular CLI
Bước 1: Mở terminal và chạy lệnh sau để tạo một Service:
ng generate service services/data
Lệnh trên sẽ tạo 2 file:
-
src/app/services/data.service.ts
-
src/app/services/data.service.spec.ts
(file test)
Bước 2: Mở file data.service.ts
, nội dung sẽ như sau:
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root', // Đăng ký Service ở cấp độ root
})
export class DataService {
constructor() { }
}
Giải thích:
-
@Injectable({ providedIn: 'root' })
giúp Service có thể được sử dụng ở bất cứ đâu trong ứng dụng mà không cần đăng ký trongproviders
. - Constructor của Service có thể chứa các logic khởi tạo nếu cần.
3. Sử dụng Service trong Component
Bước 1: Mở Component muốn sử dụng Service, ví dụ app.component.ts
.
Bước 2: Import DataService
và Inject vào Constructor của Component.
import { Component, OnInit } from '@angular/core';
import { DataService } from './services/data.service';
@Component({
selector: 'app-root',
template: `<h1>{{ message }}</h1>`,
})
export class AppComponent implements OnInit {
message: string = '';
constructor(private dataService: DataService) { }
ngOnInit() {
this.message = 'Service hoạt động!';
}
}
Giải thích:
-
private dataService: DataService
trong constructor giúp Angular tự động inject Service vào Component. - Khi Component khởi tạo (
ngOnInit()
), ta có thể gọi các phương thức của Service để lấy dữ liệu.
4. Chia sẻ dữ liệu giữa các Component bằng Service
Bước 1: Cập nhật DataService
để có một biến lưu trữ dữ liệu.
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root',
})
export class DataService {
private data: string = 'Dữ liệu ban đầu';
getData() {
return this.data;
}
setData(newData: string) {
this.data = newData;
}
}
Giải thích:
-
getData()
: Trả về dữ liệu hiện tại. -
setData(newData: string)
: Cập nhật dữ liệu.
Bước 2: Sử dụng Service trong Component 1 (component gửi dữ liệu).
-
src/app/components/component1.component.ts
import { Component } from '@angular/core';
import { DataService } from '../services/data.service';
@Component({
selector: 'app-component1',
template: `
<input [(ngModel)]="newData" placeholder="Nhập dữ liệu">
<button (click)="updateData()">Gửi dữ liệu</button>
`,
})
export class Component1 {
newData: string = '';
constructor(private dataService: DataService) { }
updateData() {
this.dataService.setData(this.newData);
}
}
Giải thích:
- Component này nhận dữ liệu từ input và gửi vào
DataService
.
Bước 3: Sử dụng Service trong Component 2 (component nhận dữ liệu).
-
src/app/components/component2.component.ts
import { Component } from '@angular/core';
import { DataService } from '../services/data.service';
@Component({
selector: 'app-component2',
template: `<p>Dữ liệu nhận được: {{ receivedData }}</p>`,
})
export class Component2 {
receivedData: string = '';
constructor(private dataService: DataService) {
this.receivedData = this.dataService.getData();
}
}
Giải thích:
- Component này gọi
getData()
từDataService
để lấy dữ liệu mới nhất.
5. Cách tốt hơn để chia sẻ dữ liệu bằng BehaviorSubject
(RxJS)
Thay vì dùng biến thông thường, ta có thể dùng BehaviorSubject
của RxJS để cập nhật dữ liệu theo thời gian thực.
Cập nhật DataService
để dùng BehaviorSubject
:
import { Injectable } from '@angular/core';
import { BehaviorSubject } from 'rxjs';
@Injectable({
providedIn: 'root',
})
export class DataService {
private dataSubject = new BehaviorSubject<string>('Dữ liệu ban đầu');
currentData = this.dataSubject.asObservable();
updateData(newData: string) {
this.dataSubject.next(newData);
}
}
Giải thích:
-
BehaviorSubject
giúp theo dõi dữ liệu và cập nhật ngay khi có thay đổi.
Component gửi dữ liệu:
this.dataService.updateData(this.newData);
Component nhận dữ liệu:
this.dataService.currentData.subscribe(data => this.receivedData = data);
Ưu điểm:
- Component luôn cập nhật dữ liệu mới nhất ngay lập tức.
- Dữ liệu đồng bộ giữa các Component.
Kết luận
Nội dung | Mô tả |
---|---|
Service là gì? | Lớp quản lý logic và dữ liệu, giúp tách biệt khỏi Component. |
Cách tạo Service | Sử dụng ng generate service services/data . |
Sử dụng Service trong Component | Inject vào Constructor của Component. |
Chia sẻ dữ liệu giữa Component | Dùng Service để lưu dữ liệu và truyền giữa các Component. |
RxJS BehaviorSubject | Giúp dữ liệu cập nhật theo thời gian thực. |
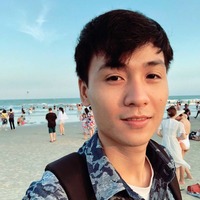
Với hơn 10 năm kinh nghiệm lập trình web và từng làm việc với nhiều framework, ngôn ngữ như PHP, JavaScript, React, jQuery, CSS, HTML, CakePHP, Laravel..., tôi hy vọng những kiến thức được chia sẻ tại đây sẽ hữu ích và thiết thực cho các bạn.
Xem thêm
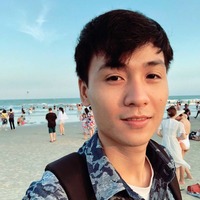
Chào, tôi là Vũ. Đây là blog hướng dẫn lập trình của tôi.
Liên hệ công việc qua email dưới đây.
lhvuctu@gmail.com