MySQL/PostgreSQL trong Node.js bằng Sequelize hoặc mysql2
1. Giới thiệu về MySQL/PostgreSQL và Sequelize
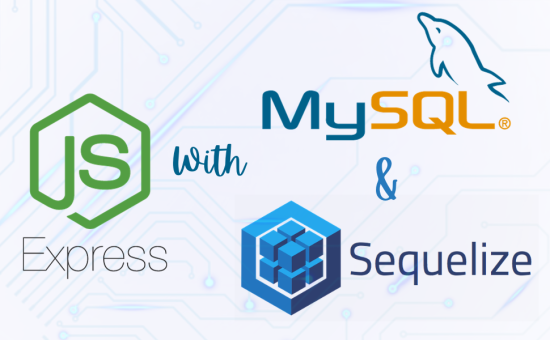
MySQL và PostgreSQL là gì?
- MySQL là hệ quản trị cơ sở dữ liệu quan hệ (RDBMS) phổ biến, nhanh, dễ sử dụng.
- PostgreSQL là RDBMS mạnh mẽ, hỗ trợ nhiều tính năng nâng cao như JSON, CTE, indexing thông minh.
Sequelize là gì?
Sequelize là một ORM (Object Relational Mapping) cho Node.js, hỗ trợ nhiều cơ sở dữ liệu, bao gồm MySQL và PostgreSQL. Nó giúp:
- Quản lý database bằng mô hình hướng đối tượng.
- Viết truy vấn dễ dàng với cú pháp JavaScript.
- Hỗ trợ migration và validation dữ liệu.
2. Cài đặt Sequelize và kết nối MySQL/PostgreSQL
a. Cài đặt Sequelize và driver tương ứng
- Nếu sử dụng MySQL, cài
mysql2
:npm install sequelize mysql2
- Nếu sử dụng PostgreSQL, cài
pg
vàpg-hstore
:npm install sequelize pg pg-hstore
3. Kết nối Sequelize với MySQL/PostgreSQL
Tạo file database.js
:
const { Sequelize } = require('sequelize');
const sequelize = new Sequelize('database_name', 'username', 'password', {
host: 'localhost',
dialect: 'mysql', // hoặc 'postgres' nếu dùng PostgreSQL
logging: false // Tắt log SQL trên console
});
// Kiểm tra kết nối
sequelize.authenticate()
.then(() => console.log('Kết nối database thành công!'))
.catch(err => console.error('Lỗi kết nối database:', err));
module.exports = sequelize;
- database_name: Tên database.
- username và password: Thông tin đăng nhập.
- dialect: Chọn mysql hoặc postgres.
4. Xây dựng Model và Migration với Sequelize
Tạo thư mục models/
và file User.js
:
const { DataTypes } = require('sequelize');
const sequelize = require('../database');
const User = sequelize.define('User', {
id: {
type: DataTypes.INTEGER,
autoIncrement: true,
primaryKey: true
},
name: {
type: DataTypes.STRING,
allowNull: false
},
email: {
type: DataTypes.STRING,
allowNull: false,
unique: true
},
age: {
type: DataTypes.INTEGER,
allowNull: false
}
}, {
timestamps: true
});
module.exports = User;
- User: Model đại diện cho bảng users.
-
timestamps: true: Tự động thêm
createdAt
vàupdatedAt
.
5. Đồng bộ hóa Model với Database
Trong database.js
, thêm:
const User = require('./models/User');
// Tạo bảng nếu chưa có
sequelize.sync()
.then(() => console.log('Database đã được đồng bộ!'))
.catch(err => console.error('Lỗi đồng bộ database:', err));
Chạy lệnh:
node database.js
→ Sequelize sẽ tự động tạo bảng users trong MySQL/PostgreSQL.
6. Thực hiện CRUD với Sequelize
Tạo file app.js
và viết các thao tác với database.
a. Thêm dữ liệu (Create)
const User = require('./models/User');
const createUser = async () => {
try {
const newUser = await User.create({
name: 'Nguyễn Văn A',
email: 'vana@example.com',
age: 25
});
console.log('Thêm user thành công:', newUser.toJSON());
} catch (error) {
console.error('Lỗi khi thêm user:', error);
}
};
createUser();
b. Lấy dữ liệu (Read)
const getUsers = async () => {
try {
const users = await User.findAll();
console.log('Danh sách users:', users.map(user => user.toJSON()));
} catch (error) {
console.error('Lỗi khi lấy danh sách users:', error);
}
};
getUsers();
c. Cập nhật dữ liệu (Update)
const updateUser = async (email, newAge) => {
try {
const updatedUser = await User.update(
{ age: newAge },
{ where: { email: email } }
);
console.log('User đã được cập nhật:', updatedUser);
} catch (error) {
console.error('Lỗi khi cập nhật user:', error);
}
};
updateUser('vana@example.com', 30);
d. Xóa dữ liệu (Delete)
const deleteUser = async (email) => {
try {
await User.destroy({ where: { email: email } });
console.log('User đã bị xóa');
} catch (error) {
console.error('Lỗi khi xóa user:', error);
}
};
deleteUser('vana@example.com');
7. Kết hợp với Express để tạo API CRUD
Tạo file server.js
:
const express = require('express');
const bodyParser = require('body-parser');
const User = require('./models/User');
const app = express();
app.use(bodyParser.json());
// API: Lấy danh sách user
app.get('/users', async (req, res) => {
const users = await User.findAll();
res.json(users);
});
// API: Thêm user mới
app.post('/users', async (req, res) => {
try {
const user = await User.create(req.body);
res.json(user);
} catch (error) {
res.status(400).json({ error: error.message });
}
});
// API: Cập nhật user theo email
app.put('/users/:email', async (req, res) => {
const { email } = req.params;
const updatedUser = await User.update(req.body, { where: { email: email } });
res.json(updatedUser);
});
// API: Xóa user theo email
app.delete('/users/:email', async (req, res) => {
const { email } = req.params;
await User.destroy({ where: { email: email } });
res.json({ message: 'User đã bị xóa' });
});
// Khởi động server
app.listen(3000, () => {
console.log('Server chạy tại http://localhost:3000');
});
8. Chạy ứng dụng
-
Khởi động MySQL hoặc PostgreSQL
service mysql start # hoặc service postgresql start
-
Chạy server
node server.js
-
Gửi request API bằng Postman hoặc cURL:
-
Lấy danh sách user:
curl -X GET http://localhost:3000/users
-
Thêm user mới:
curl -X POST http://localhost:3000/users -H "Content-Type: application/json" -d '{"name": "John", "email": "john@example.com", "age": 28}'
-
Cập nhật user:
curl -X PUT http://localhost:3000/users/john@example.com -H "Content-Type: application/json" -d '{"age": 30}'
-
Xóa user:
curl -X DELETE http://localhost:3000/users/john@example.com
-
Lấy danh sách user:
9. Kết luận
- Sequelize giúp quản lý database dễ dàng với JavaScript.
- Hỗ trợ MySQL/PostgreSQL, có thể mở rộng thêm SQLite, MSSQL.
- Kết hợp với Express, ta có thể xây dựng API CRUD nhanh chóng.
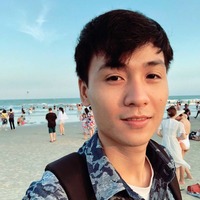
Với hơn 10 năm kinh nghiệm lập trình web và từng làm việc với nhiều framework, ngôn ngữ như PHP, JavaScript, React, jQuery, CSS, HTML, CakePHP, Laravel..., tôi hy vọng những kiến thức được chia sẻ tại đây sẽ hữu ích và thiết thực cho các bạn.
Xem thêm
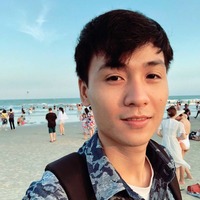
Chào, tôi là Vũ. Đây là blog hướng dẫn lập trình của tôi.
Liên hệ công việc qua email dưới đây.
lhvuctu@gmail.com