Response trong Node.js
Trong Node.js, response (phản hồi) là dữ liệu mà server gửi lại cho client sau khi nhận request. Response có thể là HTML, JSON, hoặc file tĩnh. Ngoài ra, ta có thể thiết lập HTTP headers để kiểm soát cách phản hồi hoạt động.

1. Gửi HTML trong Response
Khi client gửi request, server có thể phản hồi bằng một trang HTML.
Ví dụ: Server phản hồi HTML
const http = require("http");
const server = http.createServer((req, res) => {
res.writeHead(200, { "Content-Type": "text/html; charset=UTF-8" });
res.end(`
<html>
<head><title>Trang Chủ</title></head>
<body>
<h1>Chào mừng đến với Node.js Server</h1>
</body>
</html>
`);
});
server.listen(3000, () => {
console.log("Server chạy tại http://localhost:3000");
});
Giải thích:
-
res.writeHead(200, { "Content-Type": "text/html" })
: Thiết lập HTTP header để trình duyệt hiểu response là HTML. -
res.end()
gửi nội dung HTML về client. - Khi truy cập
http://localhost:3000
, trình duyệt sẽ hiển thị trang HTML.
2. Gửi JSON trong Response
Dữ liệu JSON thường được dùng trong API để trao đổi dữ liệu giữa client và server.
Ví dụ: Server phản hồi JSON
const http = require("http");
const server = http.createServer((req, res) => {
if (req.url === "/api/user") {
const user = {
name: "John Doe",
age: 30,
email: "john@example.com"
};
res.writeHead(200, { "Content-Type": "application/json" });
res.end(JSON.stringify(user));
} else {
res.writeHead(404, { "Content-Type": "text/plain" });
res.end("404 - Không tìm thấy dữ liệu");
}
});
server.listen(3000, () => {
console.log("Server chạy tại http://localhost:3000");
});
Giải thích:
- Nếu request đến
/api/user
, server trả về JSON chứa thông tin người dùng. -
JSON.stringify(user)
: Chuyển đổi objectuser
thành chuỗi JSON. -
Content-Type: application/json
giúp trình duyệt hiểu đây là dữ liệu JSON.
Truy cập http://localhost:3000/api/user
, bạn sẽ nhận được:
{
"name": "John Doe",
"age": 30,
"email": "john@example.com"
}
3. Gửi file tĩnh trong Response
Để phục vụ file tĩnh như HTML, CSS, JS, ảnh, ta có thể sử dụng module fs
để đọc file và gửi về client.
Ví dụ: Server gửi file HTML tĩnh
const http = require("http");
const fs = require("fs");
const path = require("path");
const server = http.createServer((req, res) => {
if (req.url === "/") {
const filePath = path.join(__dirname, "index.html");
fs.readFile(filePath, (err, data) => {
if (err) {
res.writeHead(500, { "Content-Type": "text/plain" });
res.end("Lỗi server");
} else {
res.writeHead(200, { "Content-Type": "text/html" });
res.end(data);
}
});
} else {
res.writeHead(404, { "Content-Type": "text/plain" });
res.end("404 - Không tìm thấy trang");
}
});
server.listen(3000, () => {
console.log("Server chạy tại http://localhost:3000");
});
Tạo file index.html
trong cùng thư mục:
<!DOCTYPE html>
<html lang="vi">
<head>
<meta charset="UTF-8">
<title>Trang Chủ</title>
</head>
<body>
<h1>Chào mừng đến với trang HTML từ Node.js</h1>
</body>
</html>
Truy cập http://localhost:3000/
, bạn sẽ thấy nội dung file index.html
.
4. Thiết lập HTTP Headers
Header giúp kiểm soát cách trình duyệt xử lý response. Ví dụ:
Thiết lập Content-Type
res.writeHead(200, { "Content-Type": "text/html" });
→ Trình duyệt hiểu response là HTML.
Thiết lập Cache-Control (Bộ nhớ đệm)
res.writeHead(200, { "Cache-Control": "max-age=3600" });
→ Trình duyệt sẽ lưu cache trong 1 giờ (3600 giây).
Thiết lập CORS (Chia sẻ tài nguyên giữa các nguồn khác nhau)
res.writeHead(200, { "Access-Control-Allow-Origin": "*" });
→ Cho phép tất cả website truy cập API.
5. Gửi Response với Express.js
Sử dụng http
module khá thủ công. Express.js giúp gửi response dễ dàng hơn.
Cài đặt Express
npm install express
Ví dụ: Response với Express
const express = require("express");
const app = express();
const path = require("path");
// Gửi HTML
app.get("/", (req, res) => {
res.send("<h1>Chào mừng đến với Express.js</h1>");
});
// Gửi JSON
app.get("/api/user", (req, res) => {
res.json({ name: "John", age: 30 });
});
// Gửi file tĩnh
app.get("/file", (req, res) => {
res.sendFile(path.join(__dirname, "index.html"));
});
// Thiết lập Header
app.get("/headers", (req, res) => {
res.set({
"Cache-Control": "max-age=3600",
"Access-Control-Allow-Origin": "*",
});
res.send("Header đã được thiết lập!");
});
app.listen(3000, () => {
console.log("Server Express chạy tại http://localhost:3000");
});
Giải thích:
-
res.send()
để gửi HTML hoặc văn bản. -
res.json()
để gửi dữ liệu JSON. -
res.sendFile()
để gửi file. -
res.set()
để thiết lập HTTP headers.
6. Kết luận
Tính năng | Node.js (http module) | Express.js |
---|---|---|
Gửi HTML | Dùng res.writeHead() và res.end() |
Dùng res.send() |
Gửi JSON | Dùng JSON.stringify() |
Dùng res.json() |
Gửi file | Dùng fs.readFile() |
Dùng res.sendFile() |
Thiết lập Header | Dùng res.writeHead() |
Dùng res.set() |
Nếu bạn làm một ứng dụng đơn giản, http
module là đủ. Nhưng nếu bạn cần xử lý API hoặc web phức tạp, nên dùng Express.js để tiết kiệm thời gian.
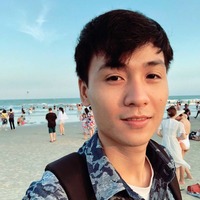
Với hơn 10 năm kinh nghiệm lập trình web và từng làm việc với nhiều framework, ngôn ngữ như PHP, JavaScript, React, jQuery, CSS, HTML, CakePHP, Laravel..., tôi hy vọng những kiến thức được chia sẻ tại đây sẽ hữu ích và thiết thực cho các bạn.
Xem thêm
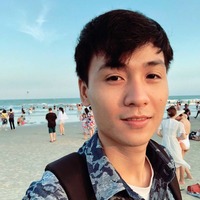
Chào, tôi là Vũ. Đây là blog hướng dẫn lập trình của tôi.
Liên hệ công việc qua email dưới đây.
lhvuctu@gmail.com