Yêu Cầu HTTP Với Requests
1. Requests Là Gì?
requests
là thư viện Python phổ biến để gửi các yêu cầu HTTP, giúp lấy dữ liệu từ web, gửi biểu mẫu, xác thực và làm việc với API một cách dễ dàng.

Cài đặt Requests:
pip install requests
Import thư viện:
import requests
2. Gửi Yêu Cầu GET
Lệnh GET
thường dùng để lấy dữ liệu từ một trang web hoặc API.
import requests
url = "https://jsonplaceholder.typicode.com/posts/1"
response = requests.get(url)
# Kiểm tra trạng thái
if response.status_code == 200:
print(response.json()) # Trả về dữ liệu dạng JSON
else:
print("Lỗi:", response.status_code)
Kết quả mẫu:
{
"userId": 1,
"id": 1,
"title": "Tiêu đề bài viết",
"body": "Nội dung bài viết..."
}
3. Gửi Yêu Cầu POST
Dùng để gửi dữ liệu đến server, ví dụ tạo mới một bài viết.
data = {
"title": "Bài viết mới",
"body": "Nội dung bài viết...",
"userId": 1
}
response = requests.post("https://jsonplaceholder.typicode.com/posts", json=data)
print(response.json()) # Xem kết quả phản hồi
4. Gửi Yêu Cầu PUT (Cập Nhật Dữ Liệu)
Cập nhật dữ liệu trên server.
data_update = {
"title": "Bài viết đã cập nhật",
"body": "Nội dung mới...",
"userId": 1
}
response = requests.put("https://jsonplaceholder.typicode.com/posts/1", json=data_update)
print(response.json())
5. Gửi Yêu Cầu DELETE
Xóa một tài nguyên trên server.
response = requests.delete("https://jsonplaceholder.typicode.com/posts/1")
print("Trạng thái:", response.status_code)
6. Thêm Tham Số Vào URL
params = {"userId": 1}
response = requests.get("https://jsonplaceholder.typicode.com/posts", params=params)
print(response.json())
7. Gửi Header Trong Request
headers = {"User-Agent": "Mozilla/5.0"}
response = requests.get("https://httpbin.org/headers", headers=headers)
print(response.json())
8. Gửi Request Với Xác Thực
auth = ("username", "password")
response = requests.get("https://httpbin.org/basic-auth/username/password", auth=auth)
print(response.status_code)
9. Xử Lý Lỗi Trong Requests
try:
response = requests.get("https://jsonplaceholder.typicode.com/posts/1", timeout=5)
response.raise_for_status() # Kiểm tra lỗi HTTP
print(response.json())
except requests.exceptions.RequestException as e:
print("Lỗi:", e)
10. Tổng Kết
Hành động | Cách sử dụng |
---|---|
Gửi GET | requests.get(url) |
Gửi POST | requests.post(url, json=data) |
Gửi PUT | requests.put(url, json=data) |
Gửi DELETE | requests.delete(url) |
Gửi tham số | requests.get(url, params=params) |
Thêm Header | requests.get(url, headers=headers) |
Xác thực | requests.get(url, auth=(user, pass)) |
Thư viện requests
giúp làm việc với HTTP dễ dàng, phù hợp để tương tác với API hoặc tự động thu thập dữ liệu web.
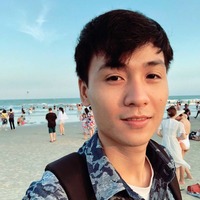
Với hơn 10 năm kinh nghiệm lập trình web và từng làm việc với nhiều framework, ngôn ngữ như PHP, JavaScript, React, jQuery, CSS, HTML, CakePHP, Laravel..., tôi hy vọng những kiến thức được chia sẻ tại đây sẽ hữu ích và thiết thực cho các bạn.
Xem thêm
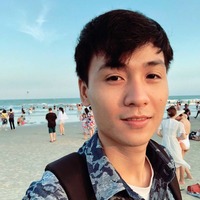
Chào, tôi là Vũ. Đây là blog hướng dẫn lập trình của tôi.
Liên hệ công việc qua email dưới đây.
lhvuctu@gmail.com